A Step-by-Step Guide to Building a CRUD API in Laravel
Jimoh Sherifdeen / September 7, 2023
2 min read
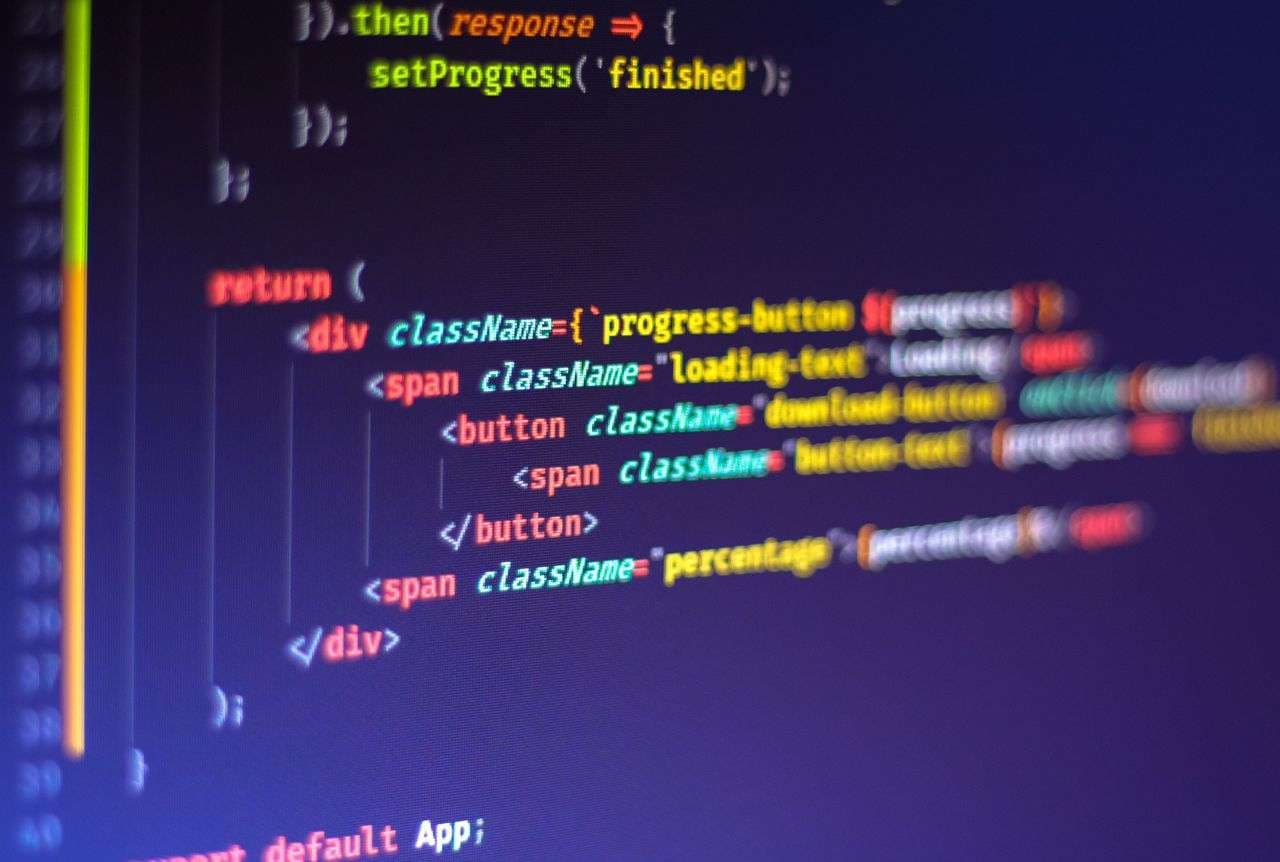
Introduction
Creating a CRUD (Create, Read, Update, Delete) API is a fundamental task in modern web development. Laravel, a popular PHP framework, simplifies this process by providing a powerful set of tools to build efficient APIs. In this article, we will walk through the steps of building a CRUD API in Laravel.
Step 5: Implementing CRUD Operations
1. Create Endpoint: Implement the store method to handle data creation.
2. Read Endpoint: Implement the index and show methods for retrieving data.
3. Update Endpoint: Implement the update method for modifying existing data.
4. Delete Endpoint: Implement the destroy method to remove data.
Step 6: Validation and Error Handling
1. Validate Requests: Utilize Laravel’s validation system to ensure data integrity
2. Error Handling: Implement error responses using JSON and appropriate HTTP status codes.
Step 7: Testing Your API
1. Write Tests: Create unit and feature tests to ensure your API functions correctly.
2. Use PHPUnit: Run tests using PHPUnit with the php artisan test command.
Step 8: API Authentication (Optional)
1. Choose Authentication Method: Decide on API authentication (e.g., Laravel Sanctum, Passport, JWT).
2. Implement Middleware: Apply authentication middleware to relevant routes.
Step 9: API Documentation (Optional)
1. Choose Documentation Tool: Select a tool like Laravel API Documentation Generator or Swagger.
2. Document Endpoints: Document your API endpoints, request, and response structures.
Step 10: Deployment
1. Set Up Server: Deploy your Laravel application on a web server (e.g., Apache, Nginx).
2. Configure Environment: Update your .env file with production settings.
Conclusion
Building a CRUD API in Laravel can seem complex at first, but with its powerful features and clear structure, the process becomes straightforward. By following this step-by-step guide, you’ve learned how to set up your environment, create database migrations, models, routes, and controllers, as well as implement CRUD operations, validation, testing, and potential authentication and documentation. This foundation will serve as a launchpad for creating robust APIs to power your web applications.