Simplifying React Context Management with BuildProviderTree
Jimoh Sherifdeen / June 1, 2024
5 min read
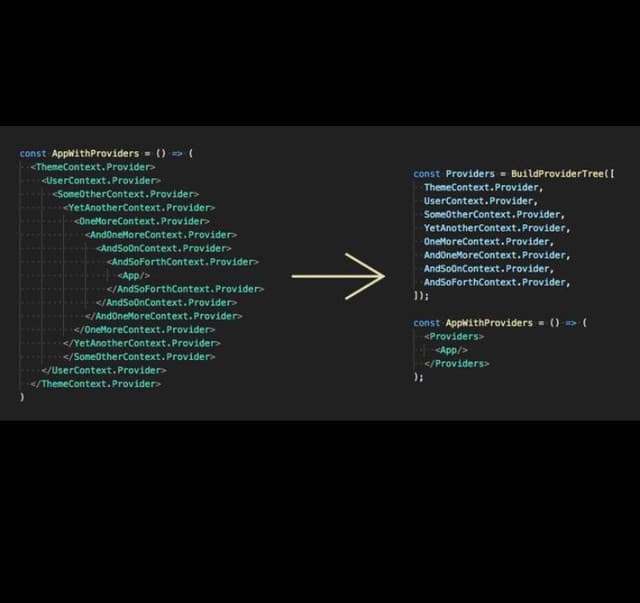
Introduction
Managing multiple context providers in a React application can quickly become a complex and cumbersome task. Nesting providers manually often leads to tangled and hard-to-maintain code. Recently, I stumbled upon an elegant solution to this problem, and I’d love to share it with you. This solution not only simplifies the management of context providers but also resonates strongly with the principles of clean code.
The Problem
In a typical React application, you might have several contexts to manage different pieces of state. For example, you might have contexts for user authentication, theme settings, application settings, and more. Manually nesting these providers can look something like this:
<ThemeProvider>
<AuthProvider>
<SettingsProvider>
<App />
</SettingsProvider>
</AuthProvider>
</ThemeProvider>
As the number of providers grows, this approach becomes increasingly unmanageable and error-prone.
The Solution: BuildProviderTree
To tackle this issue, we can use a function called BuildProviderTree. This utility function dynamically combines multiple providers into a single, clean component, keeping your main component tree readable and easy to maintain.
Here’s the implementation of BuildProviderTree:
const BuildProviderTree = (providers) => {
if (providers.length === 1) {
return providers[0];
}
const A = providers.shift();
const B = providers.shift();
return BuildProviderTree([
({ children }) => (
<A>
<B>
{children}
</B>
</A>
),
...providers,
]);
};
For a providers with props:
const BuildProviderTree = (providers) => {
if (providers.length === 1) {
const { component: Provider, props } = providers[0];
return ({ children }) => <Provider {...props}>{children}</Provider>;
}
const [{ component: A, props: propsA }, { component: B, props: propsB }] = providers.splice(0, 2);
return BuildProviderTree([
{
component: ({ children }) => (
<A {...propsA}>
<B {...propsB}>
{children}
</B>
</A>
),
props: {}
},
...providers,
]);
};
Explanation
This function recursively combines an array of providers into a single component. It works by taking two providers at a time, wrapping them, and then continuing to combine the remaining providers.
Process:
If only one provider remains, return it and it's props.
Remove the first two providers from the array.
Create a new provider component that nests the second provider inside the first.
Recursively call BuildProviderTree with the new nested provider and the remaining providers.
Example Usage
Let’s see how you can use this function in a real-world scenario. Suppose we have three context providers: ThemeProvider, AuthProvider, and SettingsProvider.
First, define your context providers:
import React, { createContext, useState } from 'react';
// Theme context
const ThemeContext = createContext();
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
return (
<ThemeContext.Provider value={{ theme, setTheme }}>
{children}
</ThemeContext.Provider>
);
};
// Auth context
const AuthContext = createContext();
const AuthProvider = ({ children }) => {
const [user, setUser] = useState(null);
return (
<AuthContext.Provider value={{ user, setUser }}>
{children}
</AuthContext.Provider>
);
};
// Settings context
const SettingsContext = createContext();
const SettingsProvider = ({ children }) => {
const [settings, setSettings] = useState({});
return (
<SettingsContext.Provider value={{ settings, setSettings }}>
{children}
</SettingsContext.Provider>
);
};
Next, combine these providers using BuildProviderTree:
const CombinedProviders = BuildProviderTree([ThemeProvider, AuthProvider, SettingsProvider]);
const App = () => {
return (
<CombinedProviders>
<YourMainComponent />
</CombinedProviders>
);
};
Combine these providers with props using BuildProviderTree:
const providers = [
{ component: ThemeProvider, props: { theme: 'dark' } },
{ component: AuthProvider, props: { user: { name: 'John Doe' } } },
{ component: SettingsProvider, props: { settings: { language: 'en' } } }
];
const CombinedProviders = BuildProviderTree(providers);
const App = () => {
return (
<CombinedProviders>
<YourMainComponent />
</CombinedProviders>
);
};
Why This Resonates with Clean Code Principles
Readability: We keep the main component tree clean and easy to understand by abstracting the nesting logic into a utility function. This makes it easier for developers to navigate and comprehend the application's structure.
Maintainability: If you need to add or remove providers, you can do so easily without modifying the core structure of your component tree. This reduces the likelihood of introducing errors and simplifies future changes.
Reusability: The BuildProviderTree function can be reused across different parts of your application or even in different projects. This promotes the DRY (Don't Repeat Yourself) principle and encourages the use of shared utilities.
Conclusion
By leveraging BuildProviderTree, we can significantly improve how we manage multiple context providers in React applications. This approach aligns well with clean code principles, enhancing readability, maintainability, and reusability.
I first encountered this elegant solution in an insightful article by Alex Korep: Combining Many Context Providers into a Tree. I highly recommend checking it out for a deeper dive into the concept.
Happy coding! 😊✨