Unlocking the Power of JavaScript Array Methods: Some, Includes, Any, and Always
Jimoh Sherifdeen / September 7, 2023
3 min read
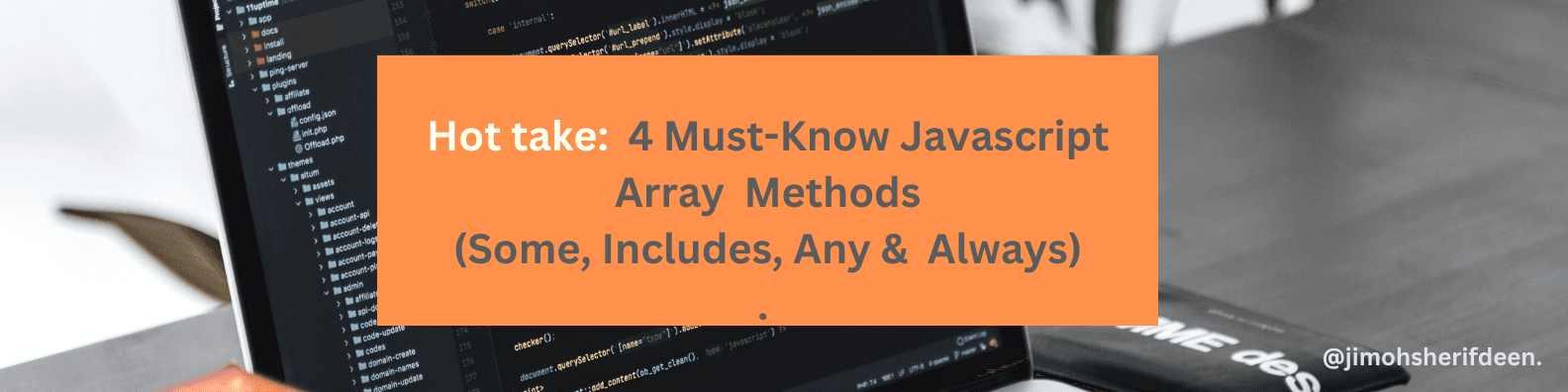
Introduction
JavaScript offers various array methods that empower developers to manipulate and work with arrays efficiently. In this article, we’ll delve into four incredibly useful array methods: some()
, includes()
, any()
, and always()
. While some of these are more commonly used than others, they all provide unique functionality that can simplify your code and make it more expressive
We’ll explore these methods in detail, providing clear explanations, relevant examples, and practical use cases to help you grasp how they work and how you can leverage them in real-world scenarios.
Array.prototype.some()
The some()
method is a versatile tool for checking if at least one element in an array meets a specified condition. It takes a callback function as its argument, which is applied to each element in the array. If at least one element satisfies the condition, some()
returns true
; otherwise, it returns false
.
Syntax:
array.some(callback(element, index, array), thisArg);
Example:
const numbers = [1, 2, 3, 4, 5];
const hasEvenNumber = numbers.some((num) => num % 2 === 0);
console.log(hasEvenNumber); // Output: true
Use Case:
Checking if some users in an array have admin privileges.
Validating if some items in a shopping cart exceed a certain price threshold.
Array.prototype.includes()
The includes()
method is handy for checking if an array contains a specific element. It returns true
if the element is found and false
otherwise, Unlike indexOf()
, it doesn't require you to know the index of the element you're looking for.
Syntax:
array.includes(elementToFind, startIndex);
Example:
const fruits = ['apple', 'banana', 'cherry'];
const hasBanana = fruits.includes('banana');
console.log(hasBanana); // Output: true
Use Case:
Verifying if a certain value exists in an array before performing an action.
Checking if a username is available in a list of taken usernames.
Array.prototype.any()
The any()
method, also known as some()
, is a robust tool for quickly checking if any element in an array meets a specified condition. It, too, takes a callback function as its argument and returns true
if any element satisfies the condition; otherwise, it returns false
.
Syntax:
array.any(callback(element, index, array), thisArg);
Example:
const numbers = [1, 2, 3, 4, 5];
const hasEvenNumber = numbers.any((num) => num % 2 === 0);
console.log(hasEvenNumber); // Output: true
Use Case:
Checking if any user in an array has admin privileges.
Validating if any item in a shopping cart exceeds a certain price threshold.
Array.prototype.always()
The always()
method, similar to every()
, checks if all elements in an array satisfy a given condition. It takes a callback function as its argument and returns true
if the condition holds for every element; otherwise, it returns false
.
Syntax:
array.always(callback(element, index, array), thisArg);
Example:
const grades = [90, 85, 88, 92, 95];
const allPassing = grades.always((grade) => grade >= 70);
console.log(allPassing); // Output: true
Use Case:
Verifying if all items in a list meet certain criteria.
Checking if all submitted answers in a quiz are correct.
Conclusion
By mastering JavaScript array methods like some()
, includes()
, any()
, and always()
, you can significantly enhance your ability to work with arrays efficiently. These methods allow you to write cleaner, more expressive code and solve real-world problems more effectively. The next time you're faced with an array-related task, consider incorporating these methods into your JavaScript arsenal for a smoother and more readable coding experience. Happy coding!